Xamarin Using MediaManager Plugin
Contents
Do you want to play media files in your Xamarin applications? With the MediaManager plugin, you can easily play audio and video files in both native and cross-platform applications. Not only can you play it, you can also customize your app as you wish.
In this article, I will show you how to add Plugin.MediaManager plugin to the project and how to use it. I will also explain the important methods in this plugin.
So, let’s started. Follow the steps below in order.
What is Plugin.MediaManager?
MediaManager plugin developed by Baseflow is a cross platform media plugin for Xamarin and Windows. It allows us to easily perform many operations such as play, pause, progress from the link address or media files on our device. You can access many features of a music player or video player application with this extension.
MediaManager plugin is one of the most useful plugins in Xamarin. I also use this plugin frequently in my projects. Just a few of the reasons I prefer it because it is small and easy to use.
1) Install MediaManager Plugin
You can download NuGet packages from both the NuGet Package Manager and the Package Manager Console in Visual Studio.
- You can install the add-in to the project by running the following line in the Package Manager Console.
Install-Package Plugin.MediaManager.Forms -Version 0.9.0
- To use it in Visual Studio, click Tools> NuGet Package Manager> Manage Packages for Solution, respectively. Then type MediaMager in the search bar and install Plugin.MediaManager.Forms to all projects. (For Xamarin.Forms)
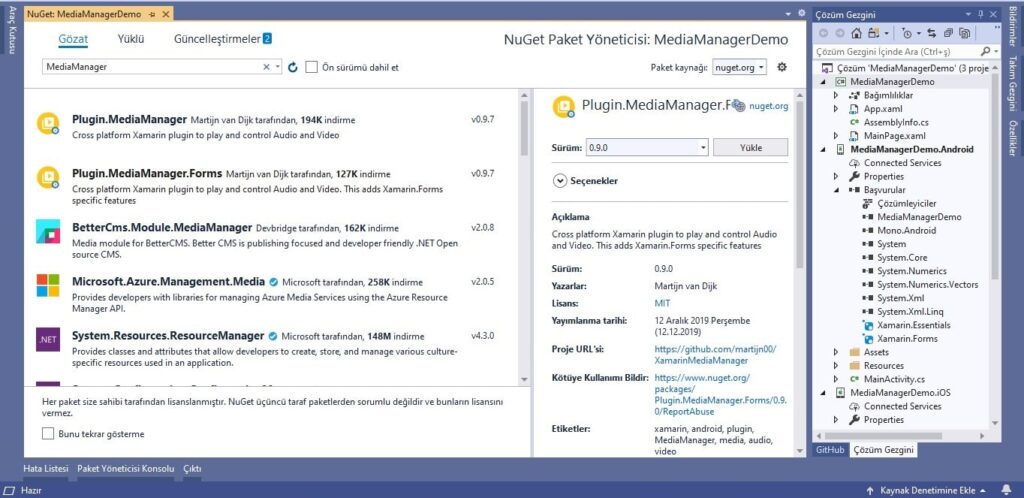
If you are getting errors with some versions of the MediaManager plug-in, install version 0.9.0.
2) Initialize MediaManager Plugin
After adding the MediaManager plug-in to the project, you cannot use it immediately. Firstly you need to launch the plugin on local platforms. After the plugin is installed, a readme.txt file containing usage instructions is opened. This file contains how to launch the plugin on local platforms.
In order to start the plugin on the Android platform, we must call the Init () method of this plugin in the MainActivity class. Add the Init () method into the onCreate () method of the MainActivity class as follows.
public class MainActivity : AppCompatActivity
{
protected override void OnCreate(Bundle savedInstanceState)
{
base.OnCreate(savedInstanceState);
SetContentView(Resource.Layout.main_activity);
CrossMediaManager.Current.Init(this);
}
}
3) Usage MediaManger Plugin
Firstly I will make a simple application that uses only Play() and Pause() methods to understand basic logic. Later I will show you how to play and control video files in your apps.
First, right click the project and add a ContentPage named MediaManagerPage.xaml. This will be the view page of the application. Then add the play.png and pause.png icons in the .Android / Resources / drawable folder. I will use these icons in view. Then open the xaml file and paste the following codes into ContentPage.
<Grid >
<Grid.RowDefinitions>
<RowDefinition Height="*"/>
<RowDefinition Height="*"/>
<RowDefinition Height="*"/>
</Grid.RowDefinitions>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="*"></ColumnDefinition>
<ColumnDefinition Width="*"></ColumnDefinition>
</Grid.ColumnDefinitions>
<ImageButton Grid.Row="1" Grid.Column="0" Source="play.png" BackgroundColor="Transparent" Clicked="PlayButtonClicked"> </ImageButton>
<ImageButton Grid.Row="1" Grid.Column="1" Source="pause.png" BackgroundColor="Transparent" Clicked="PauseButtonClicked"> </ImageButton>
</Grid>
Here I used a two-line Grid structure. And I added the icons I added to ImageButton’s Source feature. I will define the Clicked method of ImageButtons later.
After completing the view, paste the following codes into the MediaManagerPage.xaml.cs class.
public partial class MediaMangerPage : ContentPage
{
string url = "https://ia800605.us.archive.org/32/items/Mp3Playlist_555/AaronNeville-CrazyLove.mp3";
public MediaMangerPage()
{
InitializeComponent();
}
private async void PlayButtonClicked(object sender, EventArgs e)
{
await CrossMediaManager.Current.Play(url);
}
private async void PauseButtonClicked(object sender, EventArgs e)
{
await CrossMediaManager.Current.Pause();
}
}
First of all, I defined the url of a sound file as a string. Then I fill in the Clicked methods I defined in the view file here. The Play() and Pause() methods simply work this way.
The screenshot of our application will be as follows.
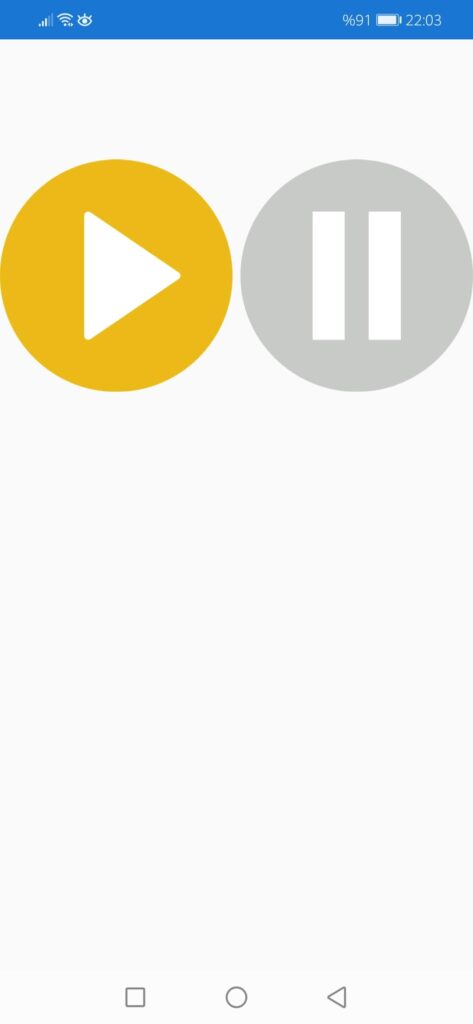
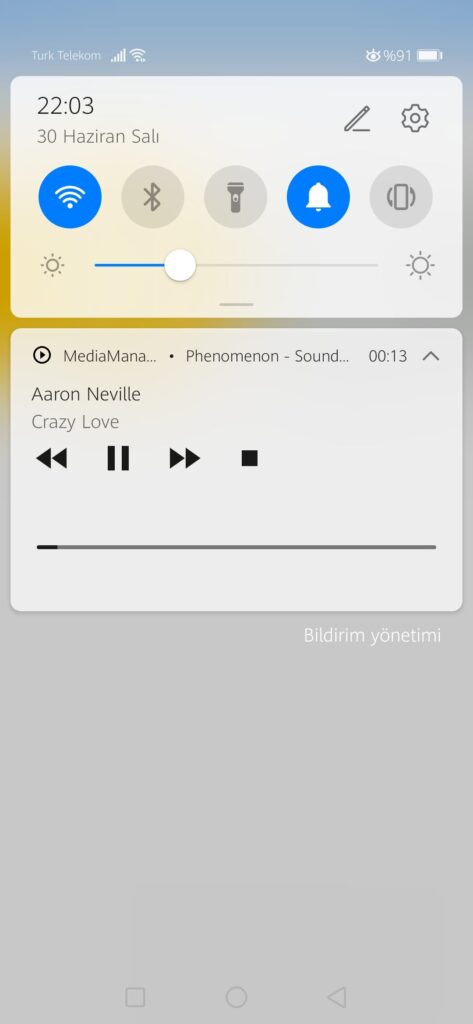
After running the application, clicking the play icon will start playing the track. Also, when you slide the notification bar down, player controls appear.
Player Methods
- Task Play(IMediaItem mediaItem); Starts playing the MediaItem
- Task Play(IMediaItem mediaItem, TimeSpan startAt, TimeSpan? stopAt = null); Starts playing the MediaItem at a given time and stops at a specific time
- Task Play(); Starts playing
- Task Pause(); Stops playing but retains position
- Task Stop(); Stops playing
- Task SeekTo(TimeSpan position); Changes position to the specified number of milliseconds from zero
Volume Properties
- event VolumeChangedEventHandler VolumeChanged; Raised when the volume changes
- int CurrentVolume { get; set; } The volume for the current MediaPlayer
- int MaxVolume { get; set; } The Maximum volume that can be used
- float Balance { get; set; } -1.0f (Left), 0.0f (Center), 1.0f (right)
- bool Muted { get; set; } True if the sound is Muted
Playback Properties
- TimeSpan StepSizeForward { get; set; } Managing the step size for the step forward function
- TimeSpan StepSizeBackward { get; set; } Managing the step size for the step backward function
- MediaPlayerState State { get; } Reading the current status of the player
- TimeSpan Position { get; } Gets the players position
- TimeSpan Duration { get; } Gets the source duration
- TimeSpan Buffered { get; } Gets the buffered time
- float Speed { get; set; } The playback speed. Can be used to make the media play slower or faster
- RepeatMode RepeatMode { get; set; }
- ShuffleMode ShuffleMode { get; set; }
Conclusions
The MediaManager plugin was simple to use. Extensive applications can be made using media files with the many methods it provides. With this plugin, you can make a complete music player application.
Moreover, you can play and control not only audio files but also video files. MediaManager plugin is also my first choice when developing a mobile application.
In this article, I explained you how to use Plugin.MediaManager plugin in Xamarin.Forms project and how to use it. I also took a closer at the important methods.
If you’re still not sure what to do, or if you got any errors, then I suggest you use the comment section below and let me know! I am here to help!
Also, share this blog post on social media and help more people learn.
Hello Serkan
I have tried copying your code in one of my .cs files in my project using VS 2019/C#/Xamarin. I get errors:
using MediaManager;
public class MainActivity : AppCompatActivity
{
protected override void OnCreate(Bundle savedInstanceState)
{
base.OnCreate(savedInstanceState);
SetContentView(Resource.Layout.main_activity);
CrossMediaManager.Current.Init(this);
}
}
Getting CS0246 type or namespace could not be found error on:
AppCompatActivity,
Bundle
Getting CS0103 name does not exist in current context
SetContentView(Resource
Getting CS1501 No overload for method init – takes 1 arguments
I want to be able to use the Play/Stop/Start/Pause functions. Also I want to use my own Buttons within my .xaml pages to call the these method, so please let me what parts of MediaManagerPage.xaml.cs I can use.
Thanks
Firstly, I suggest you to use MVVM model instead of xaml.cs in your project. You can learn how the MVVM model works with examples by reading these articles:
Xamarin.Forms MVVM Pattern
Xamarin.Forms Data Binding Context
Xamarin.Forms ListView Data Binding
For the use of MediaManager, you can check out this video:
https://www.youtube.com/watch?v=7plenjrcloM
I geting this error : “EventHandler “PlayButtonClicked” with correct signature not found in type MediaManagerPage.xaml”
Can you help me with that?
Please I noticed that media manager worked for android 8.0 downwards. From android 9.0, nothing works
Local files plays but url dont