Xamarin.Forms Cache Data with MonkeyCache Plugin
Contents
In this article, I will explain how to add a cache library to your Xamarin.Forms applications with the MonkeyCache plugin.
In mobile applications, it is important to have permanent data. Because mobile applications are not a web application, they may not always be connected to the internet. In such cases, it is better to show the data with which the user interacted with the last time rather than giving the user the error “no internet connection”.
There are several different ways to store data in mobile apps. For example, you can store your data within the application using a local database engine such as SQLite or Realm. However, if you have an application that constantly queries APIs, such as a Weather application, storing the data in the database is not a good solution.
Therefore, it is useful to use a cache library for such applications. Thus, you can keep your data in the cache for a certain period of time and show the cached data to the user even when there is no internet connection.
What is the MonkeyCache plugin?
MonkeyCache is a simple caching library for any .NET application developed by James MonteMagno. The purpose of this plugin is to allow developers to cache data for a limited time.
The data to be cached in the MonkeyCache library is stored in a Barrel. Therefore, before storing data, you must assign an application ID to Barrel.
Barrel.ApplicationId = “your_unique_name_here”;
Then add the data to the cache.
Barrel.Current.Add(key, data, expireIn);
And then get the data from the cache.
Barrel.Current.Get<Type of Data you want to get>(url);
You can remove the cached data if you wish.
Barrel.Current.EmptyAll();
In this article, I will store the data in the cache I get from the OpenWeatherMap service of the Weather application I developed previously. Follow the steps below in order.
Step 1- Install the MonkeyCache plugin in your project
First, install the MonkeyCache.FileStore NuGet package in your Xamarin.Forms project.
Type the following code in the Package Manager Console and run it. (Install and Manage NuGet Packages)
Install-Package MonkeyCache.FileStore -Version 1.5.0-beta
Alternatively, you can install this plugin from NuGet Package Manager. (Install and Manage NuGet Packages)
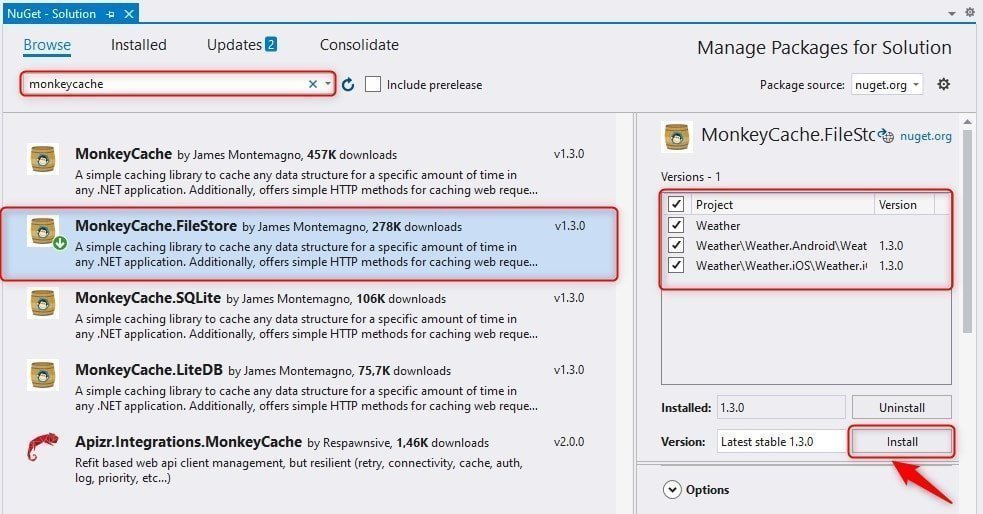
You do not need to launch it on native platforms to use the MonkeyCache plugin. Simply install the plugin in your Xamarin.Forms project.
Step 2 – Define an ApplicationId to Barrel
The data to be cached in the MonkeyCache plugin is stored in a Barrel meaning “barrel”. Therefore, you must assign the application ID to Barrel before storing data.
Assign an id value as below in the method you query for API.
public static async Task<OneCallAPI> GetOneCallAPIAsync(double lat, double lon, string units)
{
Barrel.ApplicationId = "WeatherCache";
}
Step 3 – Get data from cache if no internet connection
Now check the internet connection and whether the cached data has expired. If there is no internet connection and the data in the cache for this url has not expired, return the existing data in the cache with the Get method.
Here I used Xamarin.Essentials to check the internet connection.
public static async Task<OneCallAPI> GetOneCallAPIAsync(double lat, double lon, string units)
{
Barrel.ApplicationId = "WeatherCache";
OneCallAPI weather = new OneCallAPI();
string url = String.Format(BASE_URL2, lat, lon, units, OPENWEATHERMAP_API_KEY);
//check connectivity, if not get data from cache
if (Connectivity.NetworkAccess != NetworkAccess.Internet && !Barrel.Current.IsExpired(key: url))
{
await Task.Yield();
return Barrel.Current.Get<OneCallAPI>(key: url);
}
}
Step 4 – Add data to cache if internet connection is available
If there is an internet connection, perform an API query with this url. Then, cache the data you get from the API with the Add method.
The Add() method takes three parameters:
- Key: The URL of the web service.
- Data: The data returned by the web service.
- ExpireIn: The length of time data is kept in the cache.
public static async Task<OneCallAPI> GetOneCallAPIAsync(double lat, double lon, string units)
{
Barrel.ApplicationId = "WeatherCache";
OneCallAPI weather = new OneCallAPI();
string url = String.Format(BASE_URL2, lat, lon, units, OPENWEATHERMAP_API_KEY);
//check connectivity, if not get data from cache
if (Connectivity.NetworkAccess != NetworkAccess.Internet && !Barrel.Current.IsExpired(key: url))
{
await Task.Yield();
return Barrel.Current.Get<OneCallAPI>(key: url);
}
HttpClient httpClient = new HttpClient();
var response = await httpClient.GetAsync(url);
if (response.IsSuccessStatusCode)
{
var content = await response.Content.ReadAsStringAsync();
var posts = JsonConvert.DeserializeObject<OneCallAPI>(content);
// Add cache
Barrel.Current.Add(key: url, data: posts, expireIn: TimeSpan.FromDays(1));
weather = posts;
}
return weather;
}
Step 5 – Show your data in XAML
Finally, show the data you get from the API query in XAML. I explained how to display data in XAML with Data Binding operation using MVVM model in Weather application. I will not go back here.
After completing all the steps, run your application. There are two screenshots in my application as below.
While my device was connected to the internet, I was able to get data from the API query and cached this data to Barrel.
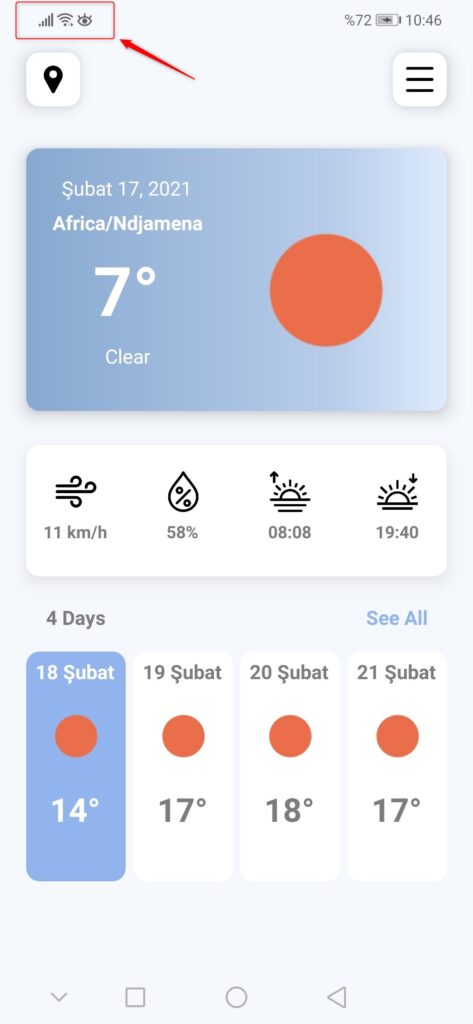
I then disconnected my device from the internet and ran the application again. This time, because there was no internet connection, the application could not query the API and showed the data in the cache.
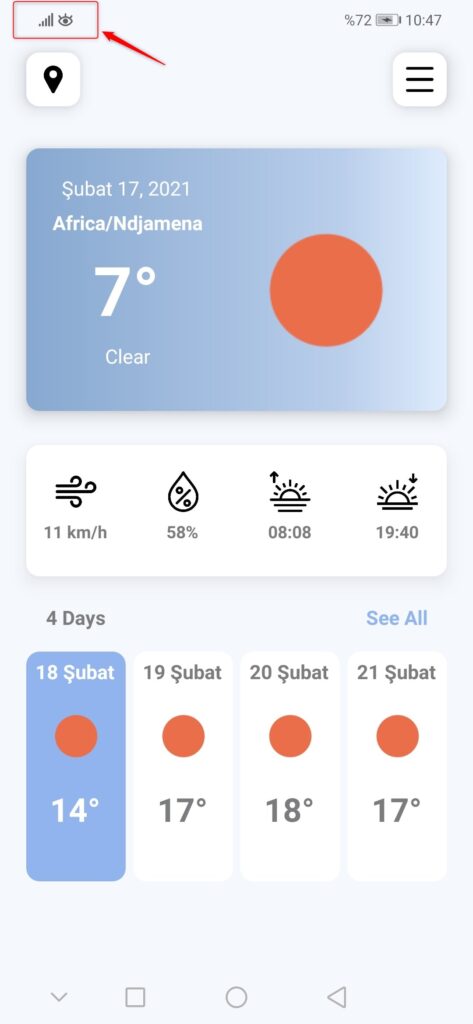
Conclusion
In this article, I explained how to add a cache library to your Xamarin.Forms applications with the MonkeyCache plugin. I hope it was helpful.
If you’re still not sure what to do, or if you got any errors, then I suggest you use the comment section below and let me know! I am here to help!
Also, share this blog post on social media and help more people learn.