Xamarin.Android Take Photo From Camera
Contents
When developing an Android application, you may sometimes need to take images from the device’s camera and use it. You may even want to store these images on the device. And by specifying the file name yourself. Or view it on another page. If you need one of these, this post is for you.
In this article, I will tell you how to take photos from the camera with MediaStore in Xamarin.Android and how to place them in ImageView. In addition, you will be able to name the images you have taken. And you will be able to store these images in the local folder. Follow the steps below to come to a conclusion. If you want to choose a photo from the gallery, check out my article called Xamarin.Android Upload Image From Gallery.
So let’s started.
1) Create Camera.xml Layout
First, you need a layout for the interface. To add a layout, go to Resources / layout in the project. Then right click on the layout folder and create a new layout by selecting Add> New Item> XML File. I named this page Camera.xml. You can name it however you want.
Add a button to open the camera and an ImageView to place the image in the camera.xml layout. Then set the id values of the button and ImageView as follows.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<Button
android:id="@+id/myButton"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="open Camera" />
<ImageView
android:src="@android:drawable/ic_menu_gallery"
android:layout_width="fill_parent"
android:layout_height="300.0dp"
android:id="@+id/imageView1"
android:adjustViewBounds="true" />
<Button
android:text="Send Message"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/sendCamera" />
</LinearLayout>
The screen output will also look like this:
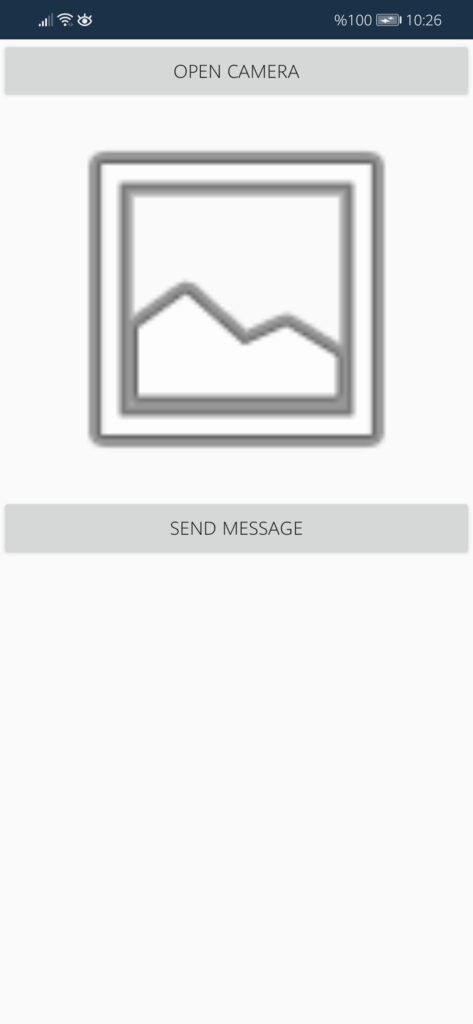
2) Create CameraActivity.cs
After adding the Camera.xml layout, you must create the activity of this layout. This activity will contain the methods of the views of the Camera.xml layout.
To add a new activity, right-click the Resources folder and select Add> New Item> Activity. I named this activity CameraActivity.cs. You can name it however you want.
Next, you have to set the layout of this activity as the Camera.xml layout. To do this, first define the necessary variables for the button and ImageView. Then define the click methods in the OnCreate () method.
Here, carefully review the onCreate () method. First, the OnCreate () method checks if there is an application using the camera with the IsThereAnAppToTakePictures () method. If not, it creates a directory for the image file with the CreateDirectoryForPictures () method.
Finally, the OnActivityResult () method displays the image taken from the camera in ImageView using Bitmap.
[Activity(Label = "CameraActivity")]
public class CameraActivity : Activity
{
private File _dir;
private File _file;
private ImageView _imageView;
protected override void OnActivityResult(int requestCode, Result resultCode, Intent data)
{
base.OnActivityResult(requestCode, resultCode, data);
// make it available in the gallery
Intent mediaScanIntent = new Intent(Intent.ActionMediaScannerScanFile);
Uri contentUri = Uri.FromFile(_file);
mediaScanIntent.SetData(contentUri);
SendBroadcast(mediaScanIntent);
// display in ImageView. We will resize the bitmap to fit the display
// Loading the full sized image will consume to much memory
// and cause the application to crash.
int height = _imageView.Height;
int width = Resources.DisplayMetrics.WidthPixels;
using (Bitmap bitmap = _file.Path.LoadAndResizeBitmap(width, height))
{
_imageView.RecycleBitmap();
_imageView.SetImageBitmap(bitmap);
string filePath = _file.Path;
}
}
protected override void OnCreate(Bundle bundle)
{
base.OnCreate(bundle);
SetContentView(Resource.Layout.Camera);
if (IsThereAnAppToTakePictures())
{
CreateDirectoryForPictures();
Button sendBtn = FindViewById<Button>(Resource.Id.sendCamera);
Button button = FindViewById<Button>(Resource.Id.myButton);
_imageView = FindViewById<ImageView>(Resource.Id.imageView1);
sendBtn.Click += SendBtn_Click;
button.Click += TakeAPicture;
}
}
private void SendBtn_Click(object sender, EventArgs e)
{
var intent = new Intent();
Bitmap bitmap = _file.Path.LoadAndResizeBitmap(50, 50);
string bit = Convert.ToString(bitmap);
intent.PutExtra("bitmap", bit);
Uri contentUri = Uri.FromFile(_file);
string uriName = Convert.ToString(contentUri);
string filePath = _file.Path;
intent.PutExtra("filepath", filePath);
intent.PutExtra("image3", uriName);
SetResult(Result.Ok, intent);
Finish();
}
private void CreateDirectoryForPictures()
{
_dir = new File(Environment.GetExternalStoragePublicDirectory(Environment.DirectoryPictures), "CameraAppDemo");
if (!_dir.Exists())
{
_dir.Mkdirs();
}
}
private bool IsThereAnAppToTakePictures()
{
Intent intent = new Intent(MediaStore.ActionImageCapture);
IList<ResolveInfo> availableActivities = PackageManager.QueryIntentActivities(intent, PackageInfoFlags.MatchDefaultOnly);
return availableActivities != null && availableActivities.Count > 0;
}
private void TakeAPicture(object sender, EventArgs eventArgs)
{
Intent intent = new Intent(MediaStore.ActionImageCapture);
_file = new File(_dir, String.Format("myPhoto_{0}.jpg", Guid.NewGuid()));
intent.PutExtra(MediaStore.ExtraOutput, Uri.FromFile(_file));
StartActivityForResult(intent, 0);
}
}
Methods, Fields and Properties
MediaStore Class
- ActionImageCapture() : It is a Standard Intent action that allows the camera app to capture and return an image.
- ExtraOutput : The name of the Intent-extra used to indicate a content resolver Uri to be used to store the requested image or video.
Environment Class
- GetExternalStoragePublicDirectory(String) : Get a top-level public external storage directory for placing files of a particular type.
- DirectoryPictures : Standard directory in which to place pictures that are available to the user.
Conclusion
Our application is ok. You can send the image you added to the camera page as PutExtra in the OnSaveClick () method. So on other pages, you can pull these extras and access the image values.
As a result, the application will work as follows. First, Camera opens with the Open Camera button. Then you take a picture. Finally, this photo you took appears in ImageView. If you wish, you can send this image to other pages with the SendMessage button.
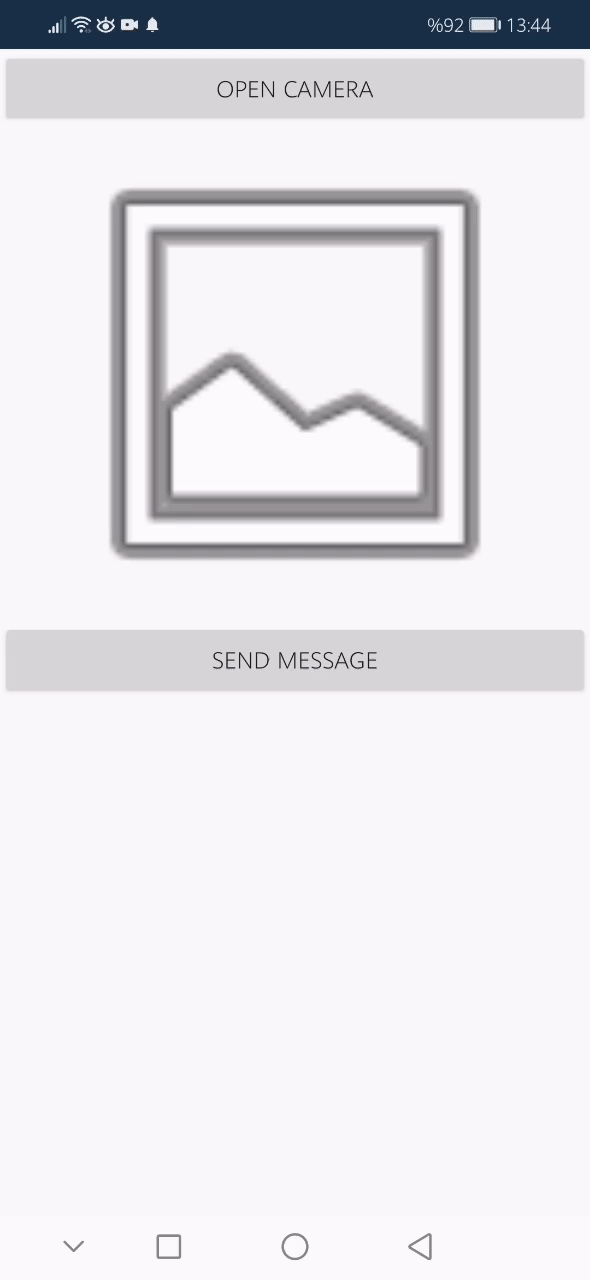
In this article, I explained how to take photos from the camera of the device with MediaStore in a Xamarin.Android application. Also to show this photo in ImageView and store it in local folder. I hope it was useful.
If you are still not sure what to do, then I suggest you use the comment section below and let me know! I am here to help!