Xamarin.Android Currency Converter
Contents
In this post, I will show you a simple application of currency converter in Xamarin.Android. It will be an application that will write the amount of foreign currency to be converted and in which currencies it is wanted to translate and return a result.
We will do this translation process by using the https://rate-exchange-1.appspot.com/ API. You can examine the site.
Currency Layout
Create an XML file named Currenct.xml by right clicking on the project and following Resources > layout > Add > New Item > XML File that named Currency.xml. Then paste these codes :
<?xml version="1.0" encoding="utf-8" ?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent">
<LinearLayout
android:orientation="vertical"
android:minWidth="25px"
android:minHeight="25px"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/linearLayout1">
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/msgText"
android:layout_marginTop="10dp"/>
</LinearLayout>
<LinearLayout
android:orientation="horizontal"
android:minWidth="25px"
android:minHeight="25px"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/linearLayout2">
<Button
android:text="$"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/currencyBtn"
/>
</LinearLayout>
</LinearLayout>
When the button is clicked in this layout, it will be translated between currencies and written into EditText. You can also use Label here.
Currency Activity
To make layout work, you need to create an Activity. To do this, right click on the Resources folder and Add > New Item > Activity named CurrencyActivity.cs
Then paste these codes :
public class CurrencyActivity : Activity
{
EditText edt;
Button currencyButton;
protected override void OnCreate(Bundle savedInstanceState)
{
base.OnCreate(savedInstanceState);
SetContentView(Resource.Layout.Currency);
edt = FindViewById<EditText>(Resource.Id.msgText);
currencyButton = FindViewById<Button>(Resource.Id.currencyBtn);
currencyButton.Click += CurrencyButton_Click;
};
}
private void CurrencyButton_Click(object sender, EventArgs e)
{
CurrencyConversion(1, "TRY", "USD");
}
private const string urlPattern = "http://rate-exchange-1.appspot.com/currency?from={0}&to={1}";
public string CurrencyConversion(decimal amount, string fromCurrency, string toCurrency)
{
string url = string.Format(urlPattern, fromCurrency, toCurrency);
string Output = edt.Text;
using (var wc = new WebClient())
{
var json = wc.DownloadString(url);
Newtonsoft.Json.Linq.JToken token = Newtonsoft.Json.Linq.JObject.Parse(json);
decimal exchangeRate = (decimal)token.SelectToken("rate");
Output = (amount * exchangeRate).ToString();
edt.Text = "1 TRY = " + Output + " USD";
return edt.Text;
}
}
}
}
Here I converted 1 Turkish Lira to Dollars. You can write the amount and currencies you want in the parameters of the method. The format is as follows : (urlPattern, fromCurrency, toCurrency).
If you want, you can send the result to another page using Bundle.
Methods
- WebClient.DownloadString() : Downloads the requested resource as a String. The resource to download may be specified as either String containing the URI or a Uri.
- String.Format() : Converts the value of objects to strings based on the formats specified and inserts them into another string.
- Object.Parse(String) : Load a JObject from a string that contains JSON.
- Object.ToString() : Returns a string that represents the current object. Object.ToString is the major formatting method in the .NET Framework. It converts an object to its string representation so that it is suitable for display.
Conclusion
We created Layout and Activity and connected them together. Our currency application is now ready. Run the app and see how it looks.
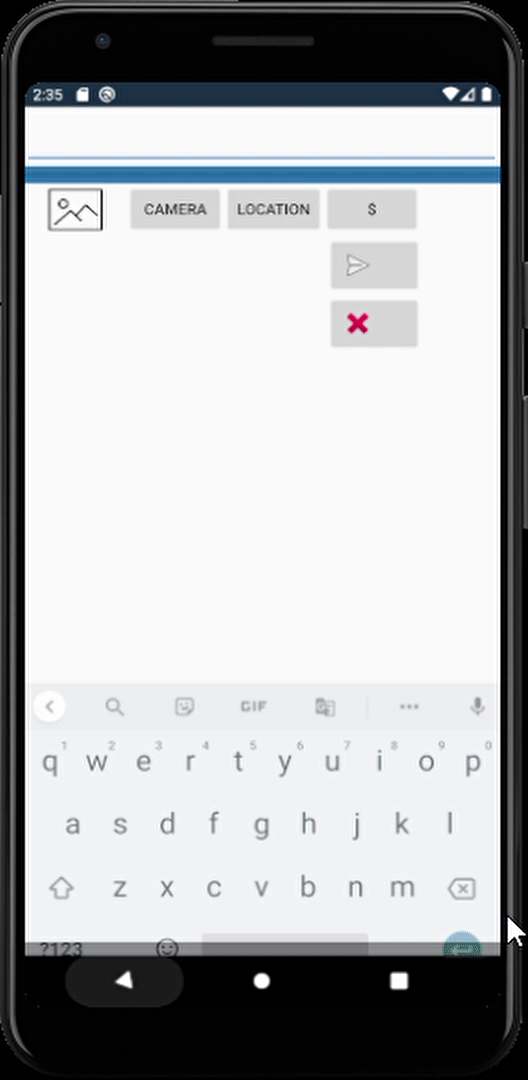