A Brief Introduction to Algorithms
Contents
Algorithms are the steps of the process that are followed in a way designed to solve a certain problem or achieve a certain goal. In other words, it means a set of rules (instructions) that define step by step how to run a job to achieve expected results.
Algorithms are a finite set of operations with a definite beginning and end. In order to reach the goal, the solutions and steps to be processed are determined and the algorithm follows these steps in order and reaches the desired solution.
Let’s look at the cooking example to better understand what the algorithm is. When you read the instructions and follow the steps in order to cook a new dish, the result is a freshly cooked dish. So the recipe has served its purpose. Similarly, algorithms also help to perform a task in programming to get the expected output.
Algorithms are often used in computer science. All programming languages are based on algorithms. Therefore, algorithms can be operated by computers through a programming language. A designed algorithm is language independent, meaning that simple instructions that can be executed in any language will have the same output as expected.
The first algorithm was presented by al-Kharizmi in his book “Hisab al-cebir ve al-mukabala”. The word algorithm was born from the European pronunciation of al-Kharizmi’s name.
What are the Features of Algorithms?
It should be noted that not all written instructions for programming are an algorithm. Some commands must have the following properties to be an algorithm:
- Should be clear and precise: An algorithm should be clear and precise. Each of its steps should be clear in all its aspects and should mean only one thing.
- Inputs should be well-defined: An algorithm’s inputs should be well-defined.
- Outputs should be well-defined: The algorithm should clearly define what output will be obtained.
- Must be finite: The algorithm must be finite, i.e. not result in an infinite loop or the like.
- Should be applicable: The algorithm should be simple, general and practical so that it can be executed according to the available resources. It should not include any future technology or anything.
- Should be language independent: The designed algorithm should be language independent. So the steps should be applicable in any language. Of course the output will be the same as expected.
How Are Algorithms Designed?
The following are needed as a prerequisite for writing an algorithm:
- Problem: A problem to be solved by an algorithm.
- Constraints: The constraints of the problem that should be considered while solving the problem.
- Input: The input to be taken to solve the problem.
- Output: The expected output when the problem is solved.
- Solution: The solution of this problem in the given constraints.
Then, the algorithm is written to solve the problem with the help of the above parameters.
Example: Calculate the sum of the 3 entered numbers
1) Providing the Prerequisites
As I mentioned above, in order to write an algorithm, its prerequisites must be met.
- Problem to be solved with this algorithm: Enter 3 numbers and calculate their sum.
- Constraints of the problem to be considered while solving the problem: Numbers must be numeric only and must not contain any other characters.
- Input to solve the problem: Three numbers to add.
- Expected output when problem is solved: The sum of three numbers taken as input.
- The solution to this problem with the given constraints: The sum of the 3 entered numbers. It can be done with the help of the ‘+’ operator or bitwise or any other method.
2) Designing the Algorithm
Now let’s design the algorithm with the help of the above prerequisites:
- START
- Define 3 integer variables, num1, num2, and num3.
- Get the 3 numbers to add as inputs in the num1, num2 and num3 variables.
- Define an integer variable (sum) to store the sum of 3 numbers.
- Add the 3 numbers and store the result in the sum variable.
- Print the value of the sum variable.
- END
3) Testing by Implementing the Algorithm
Let’s implement it in Java language to test the algorithm:
import java.util.Scanner;
class SumOfThreeNumbers{
public static void main(String args[]){
int num1,num2,num3;
System.out.println("Please enter 3 integer values");
Scanner in = new Scanner(System.in);
num1 = in.nextInt();
num2 = in.nextInt();
num3 = in.nextInt();
int sum=num1+num2+num3;
System.out.println("Result: "+sum);
}
}
The output of the program will be as follows:
Please enter 3 integer values
12
40
7
Result: 59
Analysis of Algorithms
Priori Analysis
“Priori” means “before”. Therefore, Priori analysis means checking the algorithm before its implementation. In this analysis, the algorithm is checked when written in theoretical steps. The efficiency of the algorithm is measured assuming all other factors, such as processor speed, are constant and have no effect on the application. This is usually done by the algorithm designer. The algorithm complexity is determined by this method.
Posterior Analysis
“Posterior” means “after, back”. So Posterior Analysis is the checking of the algorithm after its implementation. The algorithm is checked by implementing and running it in any programming language. This analysis is based on accuracy, space required, time spent, etc. It helps to get the real analysis report about.
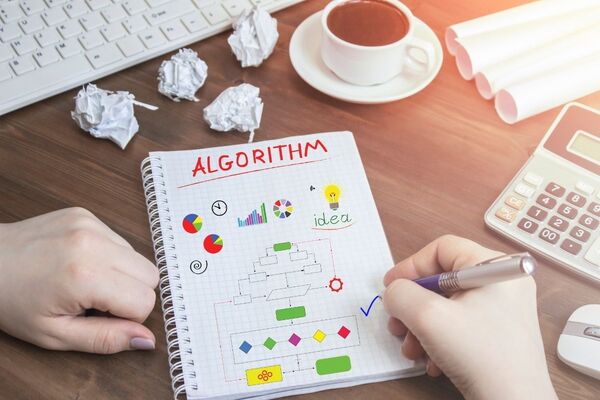
Complexity in Algorithms
- Time Factor: Time is measured by counting the number of key operations such as comparisons in the sorting algorithm.
- Space Factor: Space is measured by counting the maximum memory space required by the algorithm.
Space Complexity
The space complexity of an algorithm refers to the amount of memory that algorithm needs to execute and get the result. These inputs can be for transients or outputs.
The space complexity of an algorithm is calculated by determining the following 2 components:
- Fixed Part: The area that the algorithm absolutely needs. For example, input variables, output variables, program size, etc.
- Variable Part: The area that can be different depending on the implementation of the algorithm. For example, temporary variables, dynamic memory allocation, recursion heap space etc.
Time Complexity
The time complexity of an algorithm refers to the time it takes to execute that algorithm and get the result. This includes regular operations, conditional if-else statements, loop statements, etc. it could be.
The time complexity of an algorithm is also calculated by determining the following 2 components:
- Constant Time Part: Any instruction executed only once comes into this part. For example, input, output, if-else, key etc.
- Variable Time Part: Any command executed more than once, for example n times, comes to this part. For example loops, recursion etc.
Final Thoughts
In summary, algorithms are a set of computations that take some values as input and produce some values as output. In other words, it is the whole of the steps that receives, processes and produces outputs.
The ability to solve algorithms is the most important foundation for us to be a successful developer. In many technical interviews, the developer’s ability to solve algorithms is evaluated. Therefore, you should design algorithms well, analyze them well and reach the desired solution.
Resources:
- https://www.geeksforgeeks.org/introduction-to-algorithms/
- https://tr.wikipedia.org/wiki/Algoritma
One Comment