Animated Splash Screen
Contents
The home page takes some time to load when the mobile apps are first launched. So the user waits a bit to interact with the application. During this time, the app displays a layout to show progress or branding. These views are called Splash Screen.
We usually see plain splash screens in mobile applications. But what should you do if you want to view an animated splash screen? Here, this post is for you. Alternatively, you can make your application look more beautiful with animated Splash Screen instead of a plain splash screen.
In this post, I will take a closer at the animated splash screen construction for Xamarin.Forms and Xamarin.Android applications. I will also explain how to use Com.Airbnb.Xamarin.Forms.Lottie plugin and customize with Material Theme.
So, let’s started. Follow the steps below in order.
1) Select a Lottie Animation
Lottie animations are animations made with Adobe After Effect converted to JSON file. In this way, it is possible to use Lottie animations on many platforms. You can download many Lottie animations in JSON format on lottiefiles.com. You can also edit and add the animation of your choice before downloading it.
1 Select the animation you want to use from lottiefiles.com site and download it in JSON file format.
2 Then put this JSON file in the Android/Assests folder.
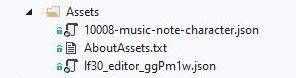
2) Install Lottie Plugin
In Xamarin.Forms, it is not possible to show an animation directly in views. Fortunately, the Com.Airbnb.Xamarin.Forms.Lottie plugin allows to show Lottie animations in Xamarin.Forms views. So, with this plugin, you can show the JSON file you downloaded. I think Com.Airbnb.Xamarin.Forms.Lottie is one of the best Xamarin plugins.
To install the Com.Airbnb.Xamarin.Forms.Lottie plugin, type the following code into the Package Manager Console and run it.
Install-Package Com.Airbnb.Xamarin.Forms.Lottie -Version 4.0.8
Alternatively, you can install it from NuGet Package Manager. Right-click the project and select Manage NuGet Package Manager for Solution. Then type Com.Airbnb.Xamarin.Forms.Lottie on the Browse tab and search And upload the plugin to the project.
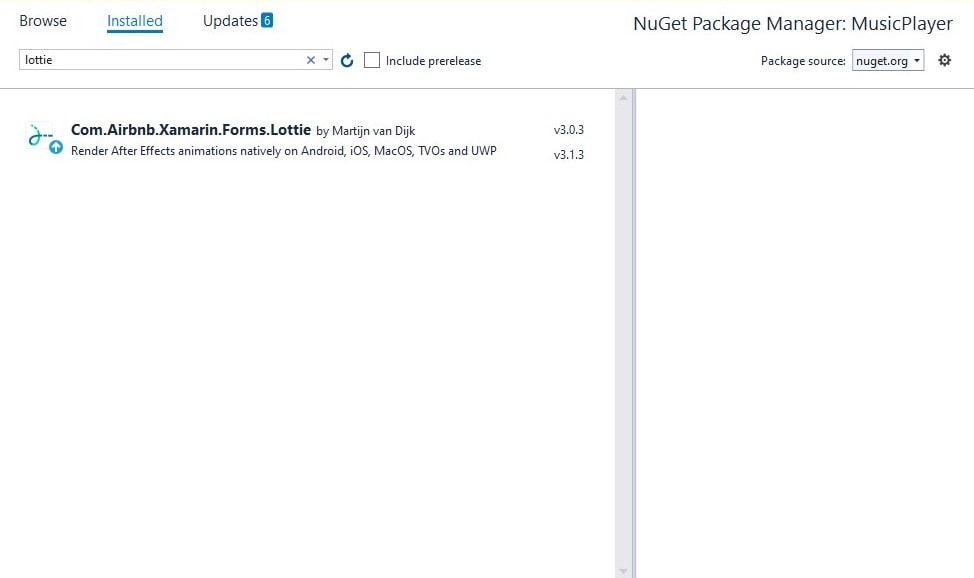
3) Create a Splash Screen Layout
Now we will create the splash layout that the user will see in this step.
1 First, go to Andorid/Resources/layout path.
2 Then right click on the layout folder and add a Layout. I named this layout SplashLayout.xml.
3 Next add a LottieAnimationView inside SplashLayout. Type the name of the JSON file in the Assests folder in the lottie_fileName property of LottieAnimationView. In this way, you can show the downloaded JSON file as an animation.
4 Finally, you can change properties such as lottie_autoPlay in LottieAnimationView to customize.
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:minWidth="25px"
android:minHeight="25px">
<com.airbnb.lottie.LottieAnimationView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:id="@+id/animation_view"
app:lottie_fileName="lf30_editor_ggPm1w.json"
app:lottie_autoPlay="true"
android:layout_centerHorizontal="true"
android:layout_alignParentBottom="true"
android:layout_marginBottom="15dp"/>
</RelativeLayout>
4) Create a Splash Theme
With the material theme, you can create a custom theme only on the Splash screen. So you can show a separate theme on the splash screen and a separate theme on the main page.
1 First, go to Android/Resources/values path.
2 Then open the styles.xml file here. The styles.xml file contains application themes.
3 Then create a custom theme called MyTheme.Splash with a <style> tags inside the <resources> tags. As follows.
<style name="MyTheme.Splash" parent ="Theme.AppCompat.Light.NoActionBar">
<item name="android:windowNoTitle">true</item>
<item name="android:windowFullscreen">true</item>
<item name="android:windowContentOverlay">@null</item>
<item name="android:windowActionBar">true</item>
</style>
You can further customize your Splash theme by changing its properties such as windowNoTitle, windowFullscreen, windowContentOverlay. I explained this topic in Material Theme. If you wish, you can get help from there.
5) Add a Splash Activity
Finally, you need an Activity to show the layout and theme. Because the application will no longer start from MainActivity. Splash activity will start first and then continue from Main Activity when the animation ends.
1 First, go to the Android folder of the project.
2 Then add an activity named SplashScreen.cs to this folder. This will be the activity before MainActivity.
3 Then change the Activity’s MainLauncher, Theme, NoHistory and Label properties as follows.
[Activity(Label = "MusicPlayer", MainLauncher = true, NoHistory = true, Theme = "@style/MyTheme.Splash")]
4 Next, let this activity inherit from the Acitivity class and the IAnimatorListener interface. And fill in the onCreate() and OnAnimationEnd() methods as below.
[Activity(Label = "MusicPlayer", MainLauncher = true, NoHistory = true, Theme = "@style/MyTheme.Splash")]
public class SplashScreen : Activity, Android.Animation.Animator.IAnimatorListener
{
public void OnAnimationCancel(Animator animation)
{
}
public void OnAnimationEnd(Animator animation)
{
StartActivity(new Intent(Application.Context, typeof(MainActivity)));
}
public void OnAnimationRepeat(Animator animation)
{
}
public void OnAnimationStart(Animator animation)
{
}
protected override void OnCreate(Bundle savedInstanceState)
{
base.OnCreate(savedInstanceState);
SetContentView(Resource.Layout.SplashLayout);
var animation = FindViewById<Com.Airbnb.Lottie.LottieAnimationView>(Resource.Id.animation_view);
animation.AddAnimatorListener(this);
}
}
Here you define an animation variable inside the onCreate() method. This animation variable will be the parameter of the OnAnimationEnd() method. Then redirect the activity to MainActivity with the OnAnimationEnd () method.
5 Finally, set the MainLauncher property of MainActivity to false. Thus, the application will not start from MainActivity.
All steps are ok. Now the application will start from the Splash activity, not the MainActivity. Then, after the animation is over, the application will continue from MainActivity. Thus, when the application is first started, the user will see an animation until MainActivty starts.
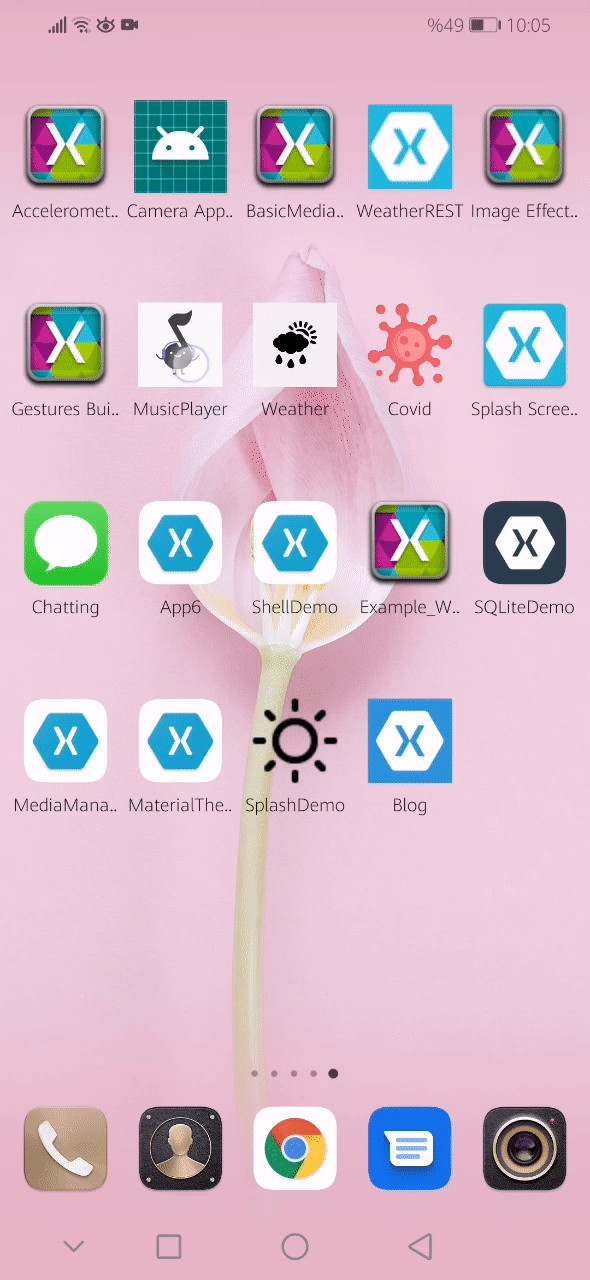
Conclusion
In summary, Splash screen is very important for a mobile application. Because one of the best ways to show branding is with the splash screen. You should definitely add splash screens to the mobile applications you develop.
In this post, I have looked a little closer at the animated login screen structure for Xamarin.Forms and Xamarin.Android applications. I also explained how to use Com.Airbnb.Xamarin.Forms.Lottie plugin and create a custom theme with Material Theme. I hope it was useful.
If you’re still not sure what to do, or if you got any errors, then I suggest you use the comment section below and let me know! I am here to help!
Also, share this blog post on social media and help more people learn.
HI,
Is it possible to achieve this on iOS?